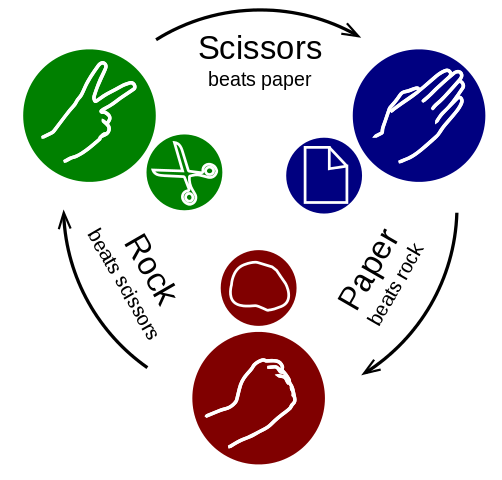
The Rock Paper Scissors game is a simple game that everyone knows how to play, and because of its simplicity, it has been used in many tutorials for teaching new programming languages. By creating a Rock Paper Scissors game, a student can grasp the concepts in a programming language such as random numbers, if statements, and “while” loop.
There are several ways to write a Rock Paper Scissors game and the basic steps are:
- The program generates a random number, 0, 1, or 2 as the computer’s choice of hand, representing Rock, Paper, Scissors. It doesn’t have to be this order and it is hidden from the user.
- The program asks the user to make a choice: Rock, Paper, or Scissors
- The program compares the computer’s hand to the user’s hand to calculate the state of the game: who has won or if the game is a draw
- If the game is a draw, then the program will repeat the steps above until either the computer or the user won the game
The most intuitive algorithm for determining the state of the game is to use string comparison. Below is a screenshot of code snippet in Python:
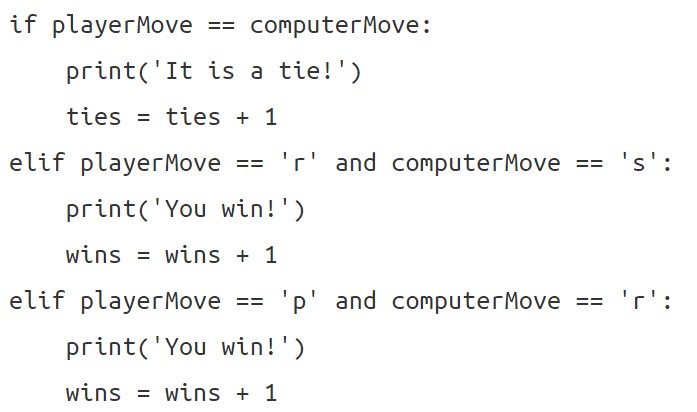
There is nothing wrong with the above approach and actually it is a good one for beginners. However, if you are more advanced in programming then you probably already see the problem of this approach: a long tedious “if” statements. As someone on StackOverflow points out that this game can be solved mathematically if Rock, Paper, and Scissors can be represented with integers like this:
Rock: 0
Paper: 1
Scissors: 2
Then you can use the modulo operator (%) to test who is the winner:
def winner(p1, p2):
if (p1 + 1) % 3 == p2:
return "Player 2 won"
elif p1 == p2:
return "It is a draw"
else:
return "Player 1 won"
This is a slick algorithm to determine the state of the game, but it is not the only one. Today, I am going to introduce another simple mathematical algorithm to solve the game:
Let’s use a different coding scheme:
Rock: 0
Paper: -1
Scissors: 1
Then we can use the following algorithm to compare the two choices of hands:
def compare_hands(hand1, hand2):
winning_hand = 2 # this represents a draw
if hand1 != hand2:
if abs(hand1) == abs(hand2):
winning_hand = max(hand1, hand2)
else:
winning_hand = min(hand1, hand2)
return winning_hand
Now, you can ask the user to choose their hands (0: Rock, -1: Paper, 1: Scissors), and the computer will generate a random number between -1 and 1, then use the above function to compare user_hand and computer_hand:
result = compare_hands(computer_hand, user_hand)
if result == 2:
print("It's a draw.")
elif result == computer_hand:
print("Computer won!")
else:
print("You won!")
Here you have it. Let me know what you think of the algorithm.
Happy programming!
How would you write this code so it’s more readable and can be understood and easily modified by other devs?